Essential JavaScript Array Methods Every Developer Should Master
Written on
Chapter 1: Understanding Array Methods
In the realm of JavaScript, comprehending array methods is vital for developers. This guide outlines essential array methods that every coder should be familiar with to enhance their programming skills.
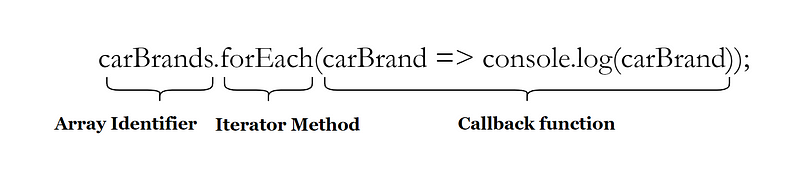
Section 1.1: The forEach() Method
The forEach() function allows you to traverse through an array and execute a callback function for each element. It's important to note that this method does not return any value.
For example, consider the following array of car brands:
const carBrands = ["mercedes", "audi", "tesla", "honda"];
carBrands.forEach(carBrand => {
console.log(carBrand);
});
// This will log each car brand to the console.
Section 1.2: The map() Method
The map() method iterates through an array, applying a callback function to each element, and produces a new array as output.
Here's how you can use it with an array of numbers:
const numbers = [1, 2, 3, 4, 5];
const new_numbers = numbers.map(number => {
return number * 2;
});
// This will return a new array: [2, 4, 6, 8, 10]
This video explains eight crucial JavaScript array methods that developers should know to enhance their coding efficiency.
Section 1.3: The filter() Method
The filter() method enables you to create a new array containing elements that meet a specific condition.
For instance, consider this array of flowers:
const flowers = ["rose", "lily", "lotus", "sunflower"];
const filtered_flowers = flowers.filter(flower => {
return flower.length <= 4;
});
// This will return: ["rose", "lily"]
Section 1.4: The reduce() Method
reduce() is a powerful method that condenses an array into a single value by executing a reducer function on each element.
Here's a simple example with numbers:
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
});
// This will yield the sum of all elements: 10
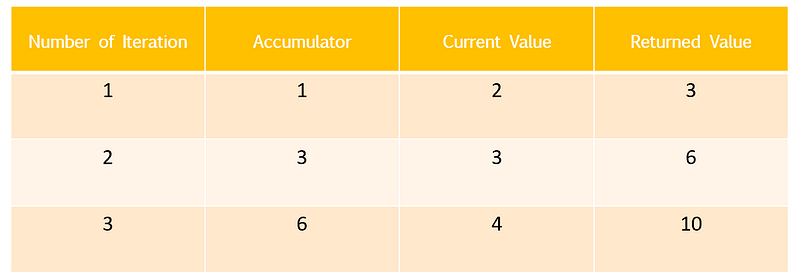
Section 1.5: The slice() Method
The slice() method extracts a segment of an array without altering the original array.
For example:
const beverages = ["tea", "coffee", "milkshake", "red bull"];
const sliced_beverages = beverages.slice(1, 3);
// This results in: ["coffee", "milkshake"]
Section 1.6: The splice() Method
Unlike slice(), the splice() method modifies the original array by removing or replacing elements.
For example:
const alphabets = ["a", "b", "c", "d"];
alphabets.splice(2, 1, "x");
// The updated 'alphabets' array will be: ["a", "b", "x", "d"]
Section 1.7: The every() Method
The every() method checks if all elements in the array satisfy a particular condition, returning a boolean value.
Here's an example:
const numbers = [10, 20, 30, 40];
numbers.every(value => value < 100); // Returns true
numbers.every(value => value > 200); // Returns false
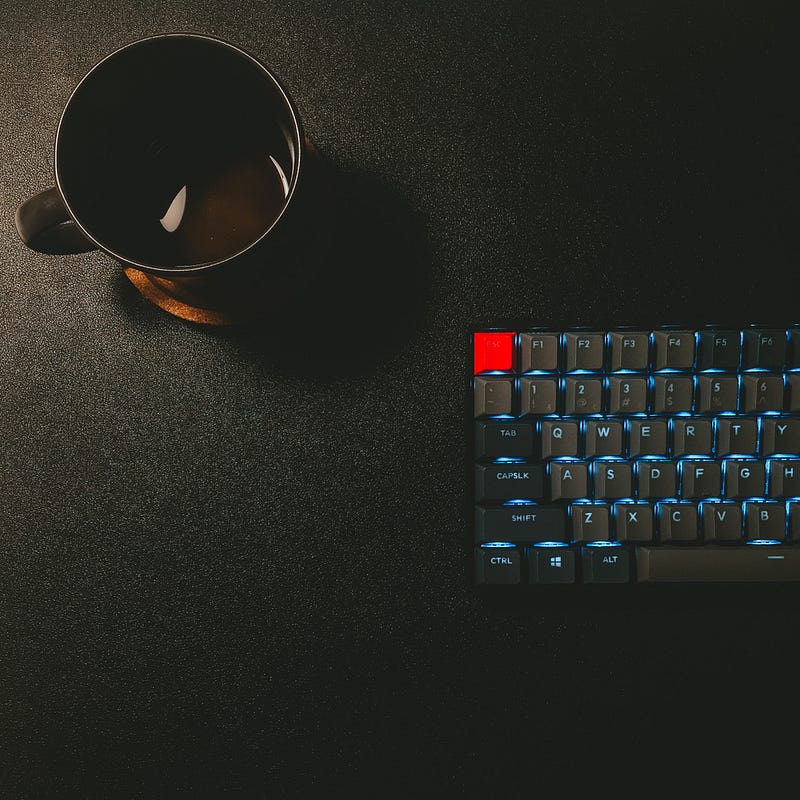
Chapter 2: Additional Resources
This video covers the most critical JavaScript array methods, providing insights that are frequently used in everyday coding.
Thank you for exploring this article! If you're new to programming or looking to learn Python, consider checking out my book, "The No Bulls**t Guide To Learning Python," linked below:
The No Bulls**t Guide To Learning Python
If you're contemplating starting your programming journey but are unsure where to begin, this guide is tailored for you.
bamaniaashish.gumroad.com
Stay connected with the Level Up Coding community for more insightful content. Follow us on Twitter, LinkedIn, or our Newsletter.