Build a Fun Quiz Game Using Vue 3 and JavaScript
Written on
Creating Your Quiz Game Project
In this guide, we will explore how to develop a quiz game utilizing Vue 3, the latest iteration of the user-friendly Vue JavaScript framework designed for crafting front-end applications.
To initiate the project, we can utilize the Vue CLI. The installation can be performed via the command:
npm install -g @vue/cli
or, if you prefer Yarn:
yarn global add @vue/cli
Once installed, we can create a new Vue project by executing:
vue create quiz-game
Make sure to select all the default settings during this setup process.
Building the Quiz Game Logic
To construct the quiz application, we will employ the following template:
<template>
<form>
<div v-if="questionIndex < questions.length">
<label>{{ question.question }}</label>
<div v-for="c of question.choices" :key="c">
<input type="radio" name="choice" v-model="answer" :value="c" />
{{ c }}
</div>
</div>
<div v-else>
<button type="button" @click="restart">Restart</button></div>
<button type="button" @click="submit">Check</button>
</form>
<p>Score: {{ score }}</p>
</template>
<script>
const questions = [
{
question: "What is American football called in England?",
choices: ["American football", "football", "Handball"],
rightAnswer: "American football",
},
{
question: "What is the largest country in the world?",
choices: ["Russia", "Canada", "United States"],
rightAnswer: "Russia",
},
{
question: "What is the 100th digit of Pi?",
choices: [9, 4, 7],
rightAnswer: 9,
},
];
export default {
name: "App",
data() {
return {
questions,
score: 0,
questionIndex: 0,
question: questions[0],
answer: "",
};
},
methods: {
submit() {
const { answer, question, questions, questionIndex } = this;
if (answer === question.rightAnswer) {
this.score++;}
if (questionIndex < questions.length - 1) {
this.questionIndex++;
this.question = { ...questions[this.questionIndex] };
}
},
restart() {
this.question = questions[0];
this.answer = "";
this.questionIndex = 0;
this.score = 0;
},
},
};
</script>
In this implementation, we create a form that displays a question only when questionIndex is less than the total number of questions. When all questions have been answered, we provide a button to restart the quiz.
The code uses radio buttons to allow users to select their answers, and we bind the selected choice to a reactive property called answer. The scoring mechanism checks if the selected answer matches the correct answer and updates the score accordingly. If there are more questions to answer, it advances to the next one. Once the quiz is complete, a restart function resets all properties to their initial state.
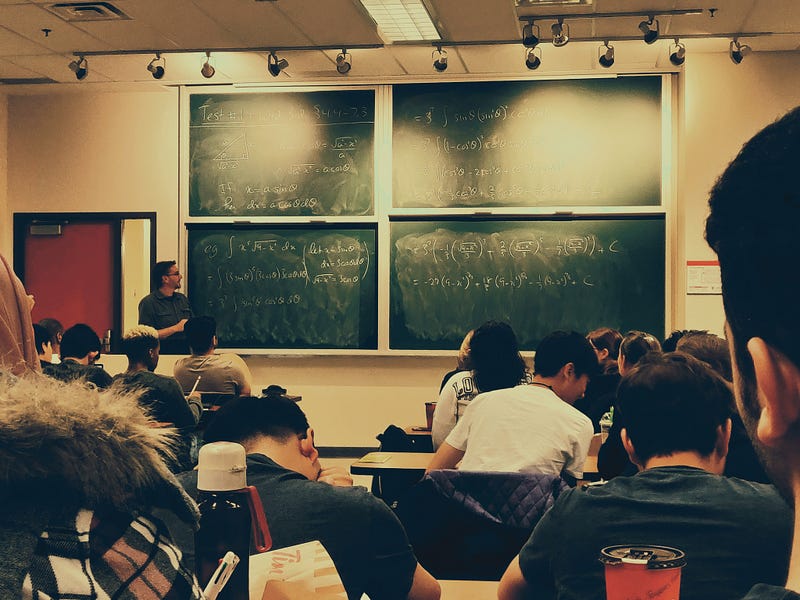
Enhancing Your Learning with Video Tutorials
To further enhance your understanding of Vue 3 and quiz game development, check out the following video tutorials:
This video covers how to build a quiz application using Vue.js, providing easy-to-follow instructions for beginners.
In this tutorial, you will learn how to create a simple quiz app with Vue 3 and Tailwind CSS, showcasing modern styling techniques.
Conclusion
Developing a quiz game using Vue 3 and JavaScript is a straightforward process that opens up numerous possibilities for interactive applications. If you enjoyed this guide, consider subscribing to our YouTube channel for more engaging content!