Mastering Programming Principles for Enhanced Code Quality
Written on
Understanding the Importance of Programming Principles
Programming encompasses the art of designing and developing software to address various technical or business challenges. Programmers are involved in the entire lifecycle of software, from designing and testing to releasing and maintaining systems. To boost productivity, they strive to create clean and maintainable code, which is where programming principles come into play.
These principles serve as widely accepted guidelines that can be applied to any software development project, regardless of the technology stack. They can act as rules that help maintain the quality of software systems. In this article, we will explore several programming principles that are vital for crafting high-quality software solutions. These principles are applicable across diverse programming projects.
Keep It Simple: Address Complex Problems with Clarity
As developers, we frequently encounter a variety of programming challenges requiring thoughtful technical architecture and coding. While some issues can be resolved with straightforward solutions, others demand intricate algorithms and complex implementations.
The YAGNI (You Aren't Gonna Need It) principle encourages developers to focus solely on the essential components required for a project. In simpler terms, there’s no need to address features or requirements that are not necessary. Similarly, the KISS (Keep It Simple, Stupid) principle advocates for designing simple, understandable architectures and code. This approach helps prevent over-engineering and promotes the use of clear, concise codebases.
However, these principles do not suggest that developers should sacrifice necessary features for simplicity. Rather, they guide programmers in achieving simplicity through thoughtful design and avoiding unnecessary complexity. For instance, the Go programming language exemplifies modern programming efficiency with its limited 25 keywords, making it a practical model for understanding YAGNI and KISS principles better.
The first video, "5 RULES to Write Better Code," elaborates on these principles and their significance in the programming landscape.
Avoiding Redundancy While Steering Clear of Over-Optimization
Repetitive code can complicate your codebase, making it harder to maintain. The DRY (Don't Repeat Yourself) principle urges developers to eliminate redundancy by creating shared code segments. For example, if two operations share similar code, consider placing that code into a single shared procedure or class.
This principle can be extended beyond individual projects. In microservice architectures, if multiple services contain duplicated code, creating a shared service or library can mitigate redundancy. Techniques like metaprogramming, including reflection, can also help reduce repetition. For instance, the Python Fire library uses reflection to transform simple code into a fully functional CLI application:
import fire
def add(a, b):
return a + b
if __name__ == '__main__':
fire.Fire()
It’s important to prevent code duplication from the outset to avoid the need for extensive refactoring later. A common pitfall is copying and modifying snippets of code without considering potential duplication. Excessive application of the DRY principle can lead to overly complex code filled with conditional statements, so it’s essential to apply it judiciously.
Prioritize Refactoring Alongside Feature Development
Even with adherence to principles like YAGNI, KISS, and DRY, code refactoring is inevitable as codebases grow. Rapidly evolving agile projects often lead to situations where initial requirements become outdated due to new feature specifications and user feedback.
The need for refactoring becomes apparent when source files are less maintainable or readable. Refactoring restructures code without adding new features or fixing bugs, which often leads programmers to postpone it since no immediate benefits are visible. Unfortunately, this can result in time-consuming refactoring down the line.
To mitigate this, establish a habit of improving your code continuously, ensuring that each iteration is better than the last. This proactive approach will save time and effort in the long run.
Architect Your Project with Modular Design
In contemporary software development, many web developers are moving away from monolithic architectures to microservices-based designs due to the latter's numerous advantages. Similarly, organizing your software projects into distinct modules, libraries, or files can facilitate better management and maintainability. For instance, the Flutter framework comprises two primary components: the Dart-based framework and the platform-specific Flutter engine, each managed in separate repositories.
Additionally, many JavaScript library developers break down their libraries into individual modules to enhance maintainability and leverage features like tree-shaking. The Firebase JavaScript SDK is a prime example of this modular approach.
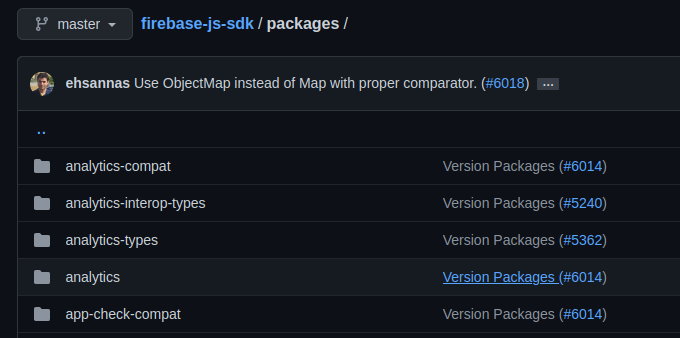
The Separation of Concerns (SoC) principle emphasizes the importance of organizing projects into multiple modules or sub-projects to improve maintainability, reusability, and independent deployment. Many layered computing components, such as internet protocols and frameworks, adhere to this pattern to reduce complexity. Consider modularizing your next project for enhanced maintainability, but be cautious about over-engineering, as excessive modularization can complicate your system.
Optimize Code Without Premature Optimization
Historically, programmers focused heavily on optimization due to limited hardware resources. For instance, the Apollo Guidance Computer operated with just 4 kilobytes of memory. Today, however, even smartphones come equipped with gigabytes of RAM, allowing for a different approach.
While performance remains vital in software development, especially for users on lower-end devices, modern developers often prioritize rapid feature delivery and user-friendly interfaces. Nonetheless, writing optimized code is crucial to ensure good performance, particularly when targeting a broad audience.
Effective optimization can be achieved through efficient algorithms, optimal data structures, and appropriate libraries. Analyzing time and space complexities can help enhance code efficiency. However, be wary of premature optimization—over-optimizing sections of code that do not require it can lead to unnecessary complexity.
When working with cloud-based applications, avoid prioritizing complex microservices over core functionalities. Strive to write optimized, hardware-friendly code, but don’t aim for perfection through over-optimization unless performance issues are evident.
The second video, "5 Ways First Principles Thinking Helps You Code Better," delves deeper into effective coding strategies and methodologies.
In conclusion, applying these programming principles can significantly enhance code quality and team productivity. Thanks for reading!