Mastering Enums in Ruby on Rails: A Comprehensive Guide
Written on
Chapter 1: Understanding Enums in Ruby on Rails
Enums are specialized data types comprising a collection of named values. Utilizing them can enhance your data management capabilities significantly.
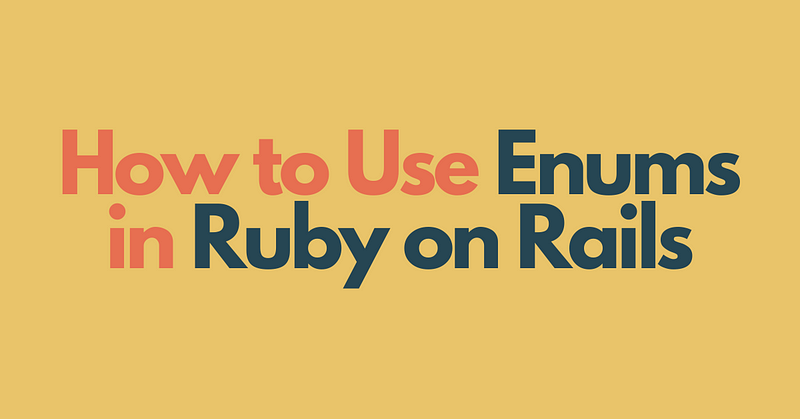
In Ruby on Rails, an enum acts as an attribute that correlates string values to integers in the database, making it possible to query these values by name. For instance, you can set up an enum for a "status" attribute with values such as pending, active, or archived. This feature was introduced in Rails 4.1.
Section 1.1: Basic Implementation of Enums
Integrating an enum into an existing model is straightforward; it involves adding an integer column to the respective table. You can create a new migration by executing the following command in your terminal:
$ bundle exec rails g migration AddStatusToUsers status:integer
This command will generate a migration file resembling this:
class AddStatusToUsers < ActiveRecord::Migration[6.0]
def change
add_column :users, :status, :integer, default: 0end
end
After that, run the migration with:
$ bundle exec rails db:migrate
In the User model, you'll need to declare the enum like this:
enum status: { pending: 0, active: 1, archived: 2 }
If you're curious about the Schema Information comments at the top of the model file, these come from the annotate gem. For further details on integrating the annotate gem into your Rails application, check out this article.
Section 1.2: Using Enum Helpers
Once your enum is set up, you can utilize various helpers that Rails provides. Let's explore a few of them.
Equality Check
To verify if a user is "active," you can use either of the following methods:
user.status == 'active'
user.active? # Returns true if user.status == 'active'
Updating the Enum
Rails also offers a helper for modifying the enum's value. Rather than using:
user.update(status: :archived)
You can simply do:
user.archived! # This is equivalent to user.update(status: :archived)
Scopes
Another useful feature of enums is the automatic generation of scopes. Instead of executing a where query such as:
User.where(status: 'active')
You can streamline this to:
User.active
Chapter 2: Common Pitfalls to Avoid
When working with enums, keep the following considerations in mind:
- Define Enums with Hashes, Not Arrays: Using an array for enum definition (e.g., enum status: [:pending, :active, :archived]) can lead to issues if you need to change the order of the values, as it may break the existing mappings. Instead, define your enums using hashes.
- Use Prefixes or Suffixes: The default behavior of enums may result in confusing scopes. It’s advisable to use either the _prefix or _suffix options. Here’s an example of a setup that enhances clarity:
enum status: {
pending: 0,
active: 1,
archived: 2
}, _prefix: true
With this configuration, all helpers will be prefixed with "status":
user.status_pending? # returns true if status is 'pending'
user.status_active! # updates the status to 'active'
User.status_archived # returns all users with status 'archived'
Chapter 3: Video Tutorials
For a deeper understanding of enums in Ruby on Rails, check out the following video tutorials.
This video, titled "How to use enums in Ruby on Rails," offers a detailed overview of implementing enums effectively.
The second tutorial, "Enums For Beginners In Ruby On Rails 7 Tutorial," provides an excellent introduction for those new to this concept.
Catalin (@cionescu1) is a Ruby on Rails consultant, founder of Organisely — A Free Appointment Management Software, and author of Modern Rails — Building a CRM in Ruby on Rails.