Creating a Free Blog with Firebase and React: A Step-by-Step Guide
Written on
Chapter 1: Introduction to Firebase
Are you interested in launching a blog without incurring costs? The appeal of a free blog that can be monetized is clear, reflected in the numerous articles dedicated to starting a blog. One often underappreciated tool for this is Firebase.
What exactly is Firebase? It’s a user-friendly platform for hosting, data storage, and website or app analytics. Firebase enables you to swiftly transform an idea into a live website without the hassle of managing backend processes.
One of its standout features is the ability to host static websites for free, offering up to 10GB of storage. This makes it an excellent option for budding bloggers who wish to explore alternatives to platforms like WordPress, Wix, or Squarespace.
Why consider alternatives like Firebase instead of established tools such as WordPress? Firebase provides greater design flexibility and functionality for your blog. To attract an audience, your blog needs to be distinctive!
Additionally, utilizing Firebase for free hosting comes with a complimentary SSL certificate, which can be costly through other hosting services.
So, what will this tutorial achieve? By the conclusion, you will have a fully functional website that retrieves data stored in Firestore and displays it on your Firebase-hosted site.
What is Firestore? Firestore is a NoSQL database service offered by Firebase. It is exceptionally user-friendly, and throughout this blog series, we will explore CRUD (Create, Read, Update, and Delete) operations using Firestore.
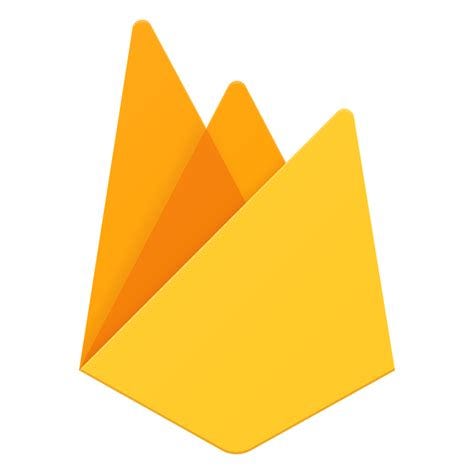
Firebase Setup
To kick off this tutorial, head over to console.firebase.google.com and create an account.
Let's start by adding a new project, which you can name anything you like.
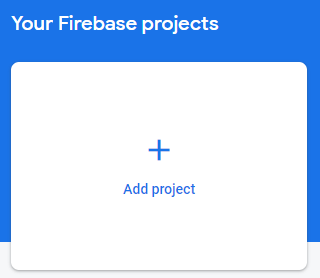
Click on ‘Add project’. Stick with the default settings and proceed until your project is established.
Next, add a web app to your project by clicking on the '+' symbol.
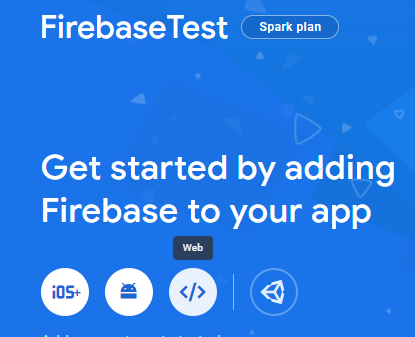
Name your app as you wish, and ensure to click ‘Add Firebase Hosting’.
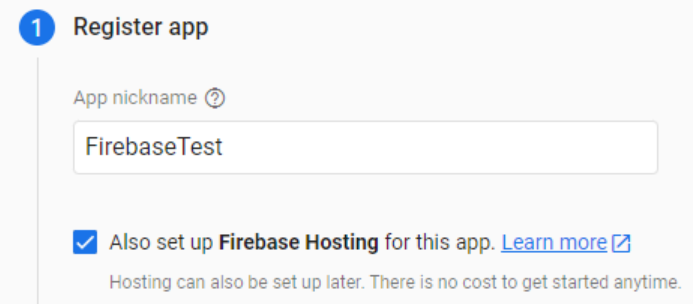
By the end of this section, your app will be created in Firebase, setting the stage for building a React app that integrates with Firebase.
Creating Your React App
Assuming you have NPM installed (if not, install it via the provided link), open your terminal and create a new folder using the command:
mkdir firebaseTest
After creating this directory, navigate into it:
cd firebaseTest
Now, let’s generate our React app with the command below, replacing ‘firebase-test’ with your desired app name:
npx create-react-app firebase-test
Once the React app is created, install Firebase and the Firebase command line tools using the following commands:
npm install firebase
npm install -g firebase-tools
By the end of this section, you should have your React app up and running, with all necessary dependencies installed. The next step involves creating the Firebase configuration file, which authenticates your app with Firebase.
Firebase Config File
With your React app set up and dependencies installed, it’s time to create the Firebase configuration file. This file contains sensitive information essential for connecting your app to the Firebase backend.
Return to the Firebase console and navigate to Project settings.
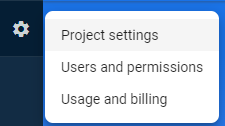
Scroll to the bottom where you’ll find ‘SDK setup and configuration details’. An SDK, or Software Development Kit, enables seamless connection between your app and Firebase. It should resemble the following structure:
const firebaseConfig = {
apiKey: “-”,
authDomain: “firebaseapp.com”,
projectId: “f”,
storageBucket: “appspot.com”,
messagingSenderId: “747”,
appId: “-”
};
(Note: Your actual appId and apiKey should remain confidential). Create a new file in the ‘src’ directory of your React project named firebase-config.js, and insert the following code, ensuring to replace the firebaseConfig variable with your SDK information:
import { initializeApp } from “firebase/app”;
import { getFirestore } from “@firebase/firestore”;
const firebaseConfig = {
apiKey: “-”,
authDomain: “fir”,
projectId: “f1”,
storageBucket: “.com”,
messagingSenderId: “47”,
appId: “-”
};
const app = initializeApp(firebaseConfig);
export const db = getFirestore(app);
In this code snippet, we import the necessary functions to establish the connection between our React app and Firebase. The initializeApp function connects your app, while getFirestore establishes the database connection.
Upon completing this section, you should have your Firebase SDK information securely integrated into firebase-config.js. Next, we’ll create the Firestore Database in Firebase.
Creating Firestore Database
Return to the Firebase console and navigate to ‘Firestore Database’.
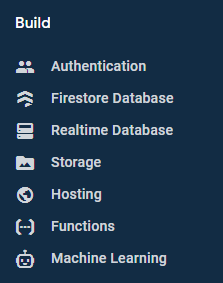
Click on ‘Firestore Database’. As previously mentioned, Firestore serves as the database for storing all your data, enabling seamless integration with your React app.
Create your database, starting it in “test-mode” for easier modifications. Firestore is document-based, meaning you'll create a collection containing various documents. For testing purposes, let’s name our collection “Posts”.
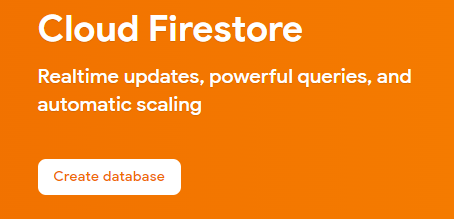
Next, we will generate some sample data to test our connection.
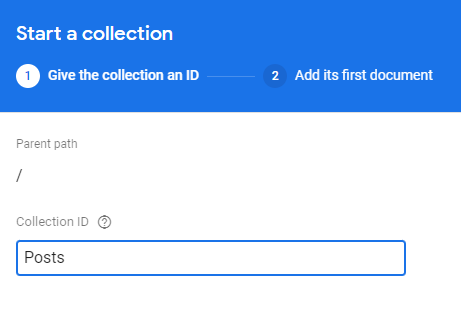
Now, we have established the connection between Firestore and our React app. The next step is to integrate everything and perform a test.
Reading Firestore Data in React App
In your src directory, locate the App.js file. Replace the existing code with the following:
import './App.css';
import { useState, useEffect } from "react";
import { db } from './firebase-config';
import { collection, getDocs } from "firebase/firestore";
function App() {
const [posts, setPosts] = useState([]);
useEffect(() => {
const postsCollectionRef = collection(db, "Posts");
const getPosts = async () => {
const data = await getDocs(postsCollectionRef);
console.log(data);
setPosts(data.docs.map((doc) => ({ ...doc.data(), id: doc.id })));
};
getPosts();
}, []);
return (
<div>
{posts.map((post) => {
return (
<div key={post.id}>
<h1>Title: {post.Title}</h1>
<h3>Description: {post.Description}</h3>
</div>
);
})}
</div>
);
}
export default App;
This code exports a function, App, which retrieves data from your Firestore Database. To run your app locally, execute the following command:
npm start
A window should open, displaying your app at http://localhost:3000/. Your website should resemble this:
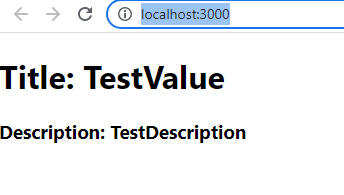
At this stage, you have a functional website running locally that retrieves data from Firebase using the credentials set up in previous sections. The next step involves hosting the website on Firebase.
Hosting on Firebase
To prepare your React app for deployment, build it using the command:
npm run build
After building your app, log into Firebase via the command line:
firebase login
Follow the on-screen prompts to complete the login process. Next, initialize your Firebase app:
firebase init
When prompted, select the features below:

Continue following the prompts, setting everything to No. When asked for your public directory, specify “build”.
Having completed the Firebase connection setup, it’s time to deploy your app. Execute the command:
firebase deploy
Your project should now be live on the web! Navigate to your website and celebrate your achievement!
Stay tuned for the upcoming parts of this series. Feel free to comment below with any questions.
Chapter 2: Tutorial Videos
To further assist you in this process, here are two helpful videos:
The first video, "Build a Blog Website - React and Firebase Tutorial," provides a comprehensive overview of creating a blog using React and Firebase.
The second video, "Build and Deploy Responsive Blog in React using Firestore | Firebase V9," guides you through the deployment of a responsive blog with Firestore.